Copyright © COSMIC Software 1999, 2003
All rights reserved.
OSMIC
Sof t ware
C
C Language manual
Rev. 1.1
Table of Contents
(i)
Preface
Chapter 1
Historical Introduction
Chapter 2
C Language Overview
C Files ......................................................................................2-1
Lines ..................................................................................2-1
Comments .......................................................................... 2-2
Trigraphs............................................................................ 2-2
Lexical Tokens.........................................................................2-3
Identifiers...........................................................................2-3
Keywords...........................................................................2-3
Constants ...........................................................................2-4
Operators and Punctuators.................................................2-4
Declarations .............................................................................2-5
Integer Types .....................................................................2-5
Bit Type .............................................................................2-7
Real Types .........................................................................2-7
Pointers ..............................................................................2-8
Arrays ................................................................................2-9
Structures ...........................................................................2-9
Unions................................................................................2-9
Enumerations ...................................................................2-10
Functions .........................................................................2-10
Chapter 3
Declarations
Integers..................................................................................... 3-2
Bits ...........................................................................................3-3
Pointers ....................................................................................3-3
Arrays.......................................................................................3-4
Modifiers..................................................................................3-5
Structures ................................................................................. 3-8
Unions ....................................................................................3-10
Enumerations .........................................................................3-10
Functions................................................................................3-11
Storage Class.......................................................................... 3-13
Typedef ..................................................................................3-15
(ii)
Variable Scope ....................................................................... 3-15
Absolute Addressing.............................................................. 3-16
Chapter 4
Expressions
Variables .................................................................................. 4-2
Constants ................................................................................. 4-2
Strings ...................................................................................... 4-5
Sizeof ....................................................................................... 4-7
Operators ................................................................................. 4-7
Arithmetic operators .......................................................... 4-8
Bitwise operators ............................................................... 4-9
Boolean operators ............................................................ 4-10
Assignment operators ...................................................... 4-11
Addressing operators ....................................................... 4-13
Function call operator...................................................... 4-14
Conditional operator ........................................................ 4-16
Sequence operator ........................................................... 4-17
Conversion operator ........................................................ 4-17
Priorities................................................................................. 4-19
Chapter 5
Statements
Block statement ....................................................................... 5-2
Expression statement ............................................................... 5-2
If statement .............................................................................. 5-3
While statement ....................................................................... 5-5
Do statement ............................................................................ 5-6
For statement ........................................................................... 5-7
Break statement ....................................................................... 5-9
Continue statement ................................................................ 5-10
Switch statement .................................................................... 5-11
Goto statement ....................................................................... 5-13
Return statement .................................................................... 5-14
Chapter 6
Preprocessor
Macro Directives ..................................................................... 6-2
Hazardous Behaviours....................................................... 6-5
Predefined Symbols........................................................... 6-7
Conditional Directives ............................................................. 6-8
Control Directives.................................................................. 6-10
(iii)
#include ...........................................................................6-10
#error ............................................................................... 6-10
#line ................................................................................. 6-10
#pragma ...........................................................................6-11
© Copyright 2003 by COSMIC SoftwarePreface-1
Preface
Chapter 1, “Historical Introduction”
Chapter 2, “C Language Overview
”
- file structure, separate compile
- character set (trigraph, logical lines, preprocessor)
- naming, identifiers, keywords, operators, punctuation
- C objects (simple, aggregate)
- functions
Chapter 3, “Declarations
”
- syntax for each kind of object
- modifiers const, volatile
- extra modifiers for pointers
- function declaration, arguments and local variables
- classes and scope
- type redeclaration (typedef)
Chapter 4, “Expressions
”
- identifiers, operators and constants
- operators behaviour
- conversions, explicit, implicit
Chapter 5, “Statements
”
- description of each statement
Chapter 6, “Preprocessor
”
- description of each directives
- traps and solutions
- pragmas and their usage
© Copyright 2003 by COSMIC Software
CHAPTER
Historical Introduction 1-1
1
Historical Introduction
The C language was designed in 1972 at the Bell Lab’s by Denis
Ritchie, mainly to rewrite the UNIX operating system in a portable way.
UNIX was written originally in assembler and had to be completely
rewritten for each new machine. The C language was based on previous
languages named B and BCPL, and is logically called C to denote the
evolution.
The language is described in the well known book “The C Program-
ming Language” published in 1978, whose “Appendix A” has been used
for many years as the de facto standard. Because of the growing pop-
urality (The popularity) of UNIX and of the C language (growing), sev-
eral companies started to provide C compilers outside of the UNIX
world, and for a wide range of processors, microprocessors, and even
micro-controllers.
The success of C for those small processors is due to the fact that it is
not a high level language, as PASCAL or ADA, but a highly portable
macro assembler, allowing with the same flexibility as assembler
almost the same efficiency.
The C language has been normalized from 1983 to 1990 and is now an
ANSI/ISO standard. It implements most of the usefull extentions added
to the original language in the previous decade, and a few extra features
allowing a more secured behaviour.
1-2 Historical Introduction © Copyright 2003 by COSMIC Software
1
The ANSI/ISO standard distinguishes two different environments:
•A hosted environment describing native compilers, whose target
processor uses a specific operating system providing disks file
handling, and execution environments.
•A freestanding environment describing a cross compiler, whose
target processor is generally an embedded microprocessor or a
micro-controller without any operating system services. In other
words, everything has to be written to perform the simplest opera-
tion.
On a native system, the same machine is used to create, compile and
execute the C program. On an embedded system, the target application
has no disk, no operating system, no editor and cannot be used to create
and compile the C program. Another machine has to be used to create
and compile the application program, and then the result must be trans-
ferred and executed by the target system. The machine used to create
and compile the application is the Host system, and the machine used to
execute the application is the Ta r g e t system.
COSMIC compilers are Cross compilers and conform to the Freestand-
ing environment of the ANSI/ISO standard. They also implement
extensions to the standard to allow a better efficiency for each specific
target processor.
An evolution from the older C89 standard was introduced in 1999.
Referred to as C99 (orC9X), the new standard has added a few new
types and constructs. Some of these features have been used in COS-
MIC compilers as C99 conformant extensions to the C89 standard.
© Copyright 2003 by COSMIC Software
CHAPTER
C Language Overview 2-1
2
C Language Overview
A C program is generally split in to several files, each containing a part
of the text describing the full application. Some parts may be already
written and used from libraries. Some parts may also be written in
assembler where the C compiler is not efficient enough, or does not
allow a direct access to some specific resources of the target processor.
C Files
Each of these C files has to be compiled, which translates the C text file
to a relocatable object file. Once all the files are compiled, the linker is
used to bind together all the object files and the required libraries, in
order to produce an executable file. Note that this result file is still in an
internal format and cannot be directly transferred
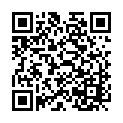
Continue reading on your phone by scaning this QR Code
Tip: The current page has been bookmarked automatically. If you wish to continue reading later, just open the
Dertz Homepage, and click on the 'continue reading' link at the bottom of the page.